Learn Spring Security
Contents
Enable Basic Authentication
Basic Auth is an authentication mechanism for any HTTP user agents like browser or REST clients like Postman to provide username and password when making a request. It is stateless where each request must contain Authorization header with value in the format Basic <Credentials>, where Credentials is the base64 encoding of username and password joined by a single colon (username:password).
Configure HttpSecurity
We can customise the Spring Security behaviour to use only Basic Authentication and ignore form based login. We can do so by configuring web based security for all http requests using HttpSecurity. We will then use it to build and register SecurityFilterChain bean inside a @Configuration class as below:
@Configuration
public class ApiSecurityConfig {
@Bean
public SecurityFilterChain apiFilterChain(HttpSecurity http) throws Exception {
http
.authorizeRequests(auth -> auth
.anyRequest().authenticated()
)
.httpBasic();
return http.build();
}
}
Here we have configured HttpSecurity to authenticate any request using Basic Auth. It might sound like the default behaviour we saw earlier. But configuring HttpSecurity explicitly with httpBasic() tells Spring Security to choose only BasicAuthenticationEntryPoint as the default EntryPoint and ignore LoginUrlAuthenticationEntryPoint.
Restart the application and access the ListCourses API from the browser. It will respond with 401 Unauthorised instead of 302 Found redirect error status. Also it will enable the browser to stay on the same page and prompt us for username and password rather than redirecting to login page. Once we enter the credentials we can see the list of courses.
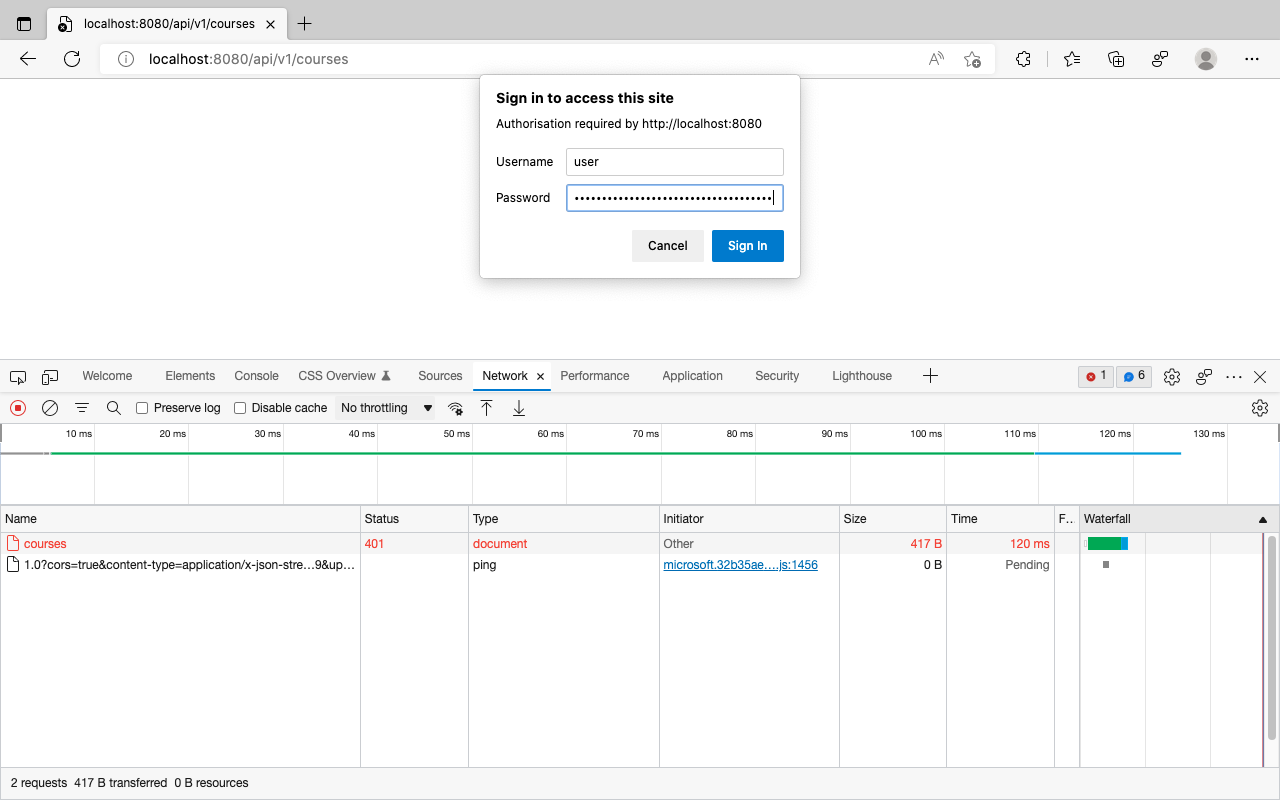
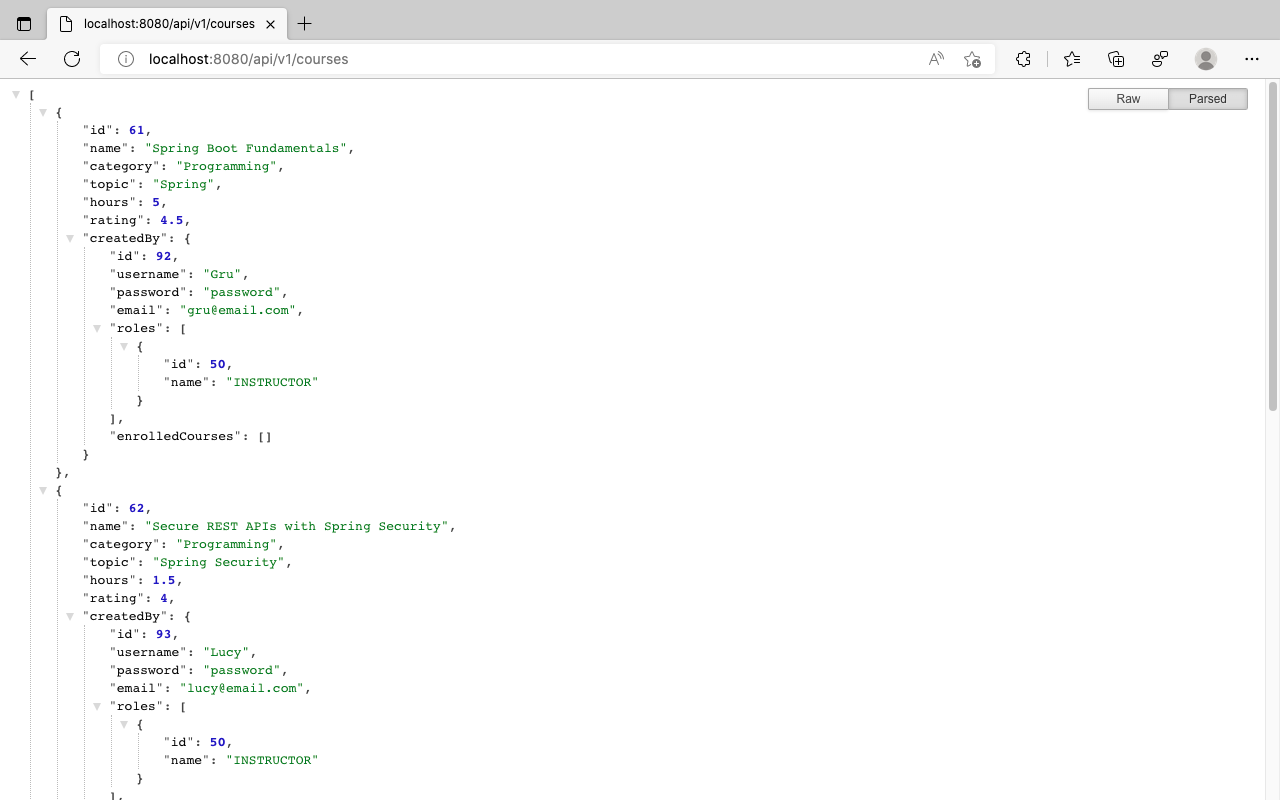