Learn Spring Security
Contents
Install Spring Security
Let's add Spring Security to the application by adding spring-boot-starter-security dependency to the pom.xml as below:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Adding any framework doesn't normally have any immediate effect until we provide the corresponding configuration. But by just adding Spring Security dependency, it secures all the resources (incl. static resources) by default. Restart the application and send a GET request to ListCourses API in Postman, instead of receiving the list of courses we will receive 401 Unauthorized error.
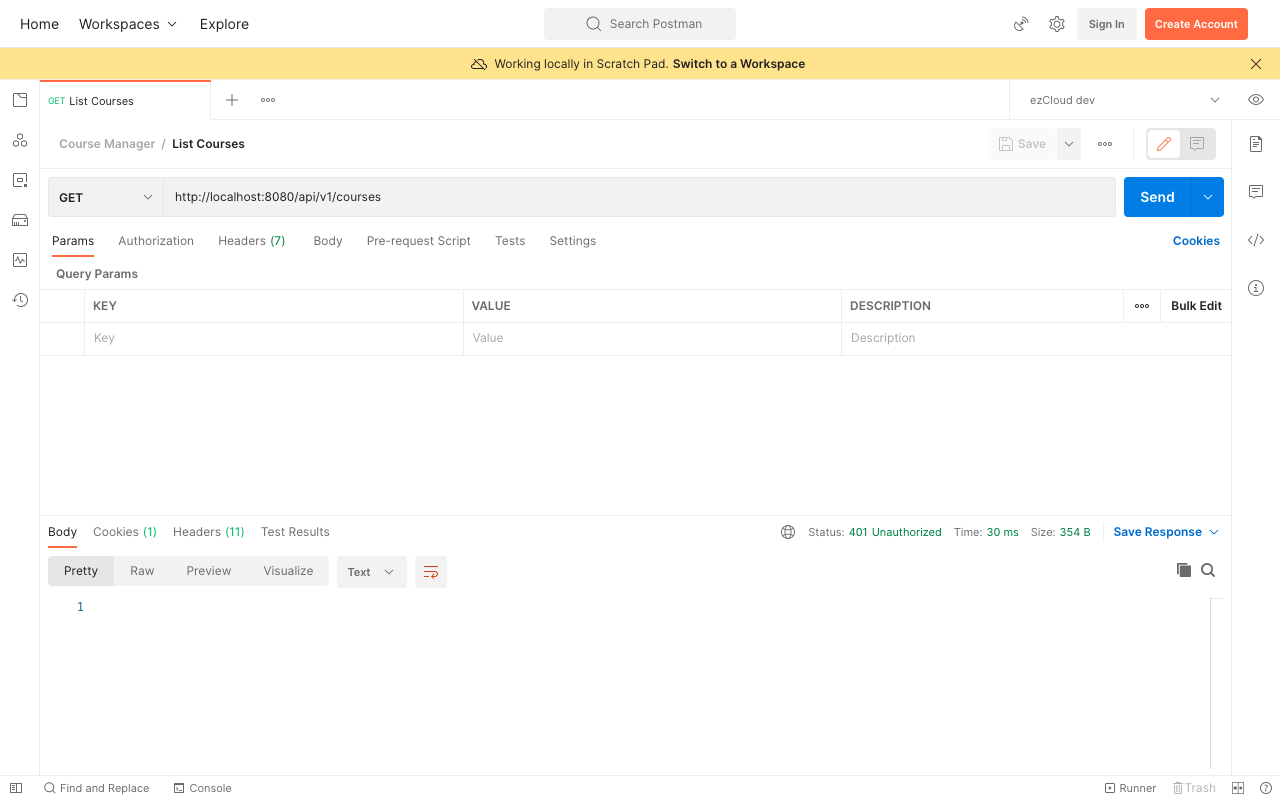
Now let's access the same API from the browser, instead of getting 401 Unauthorized error we are redirected to a Login page which was not created by us in the application.
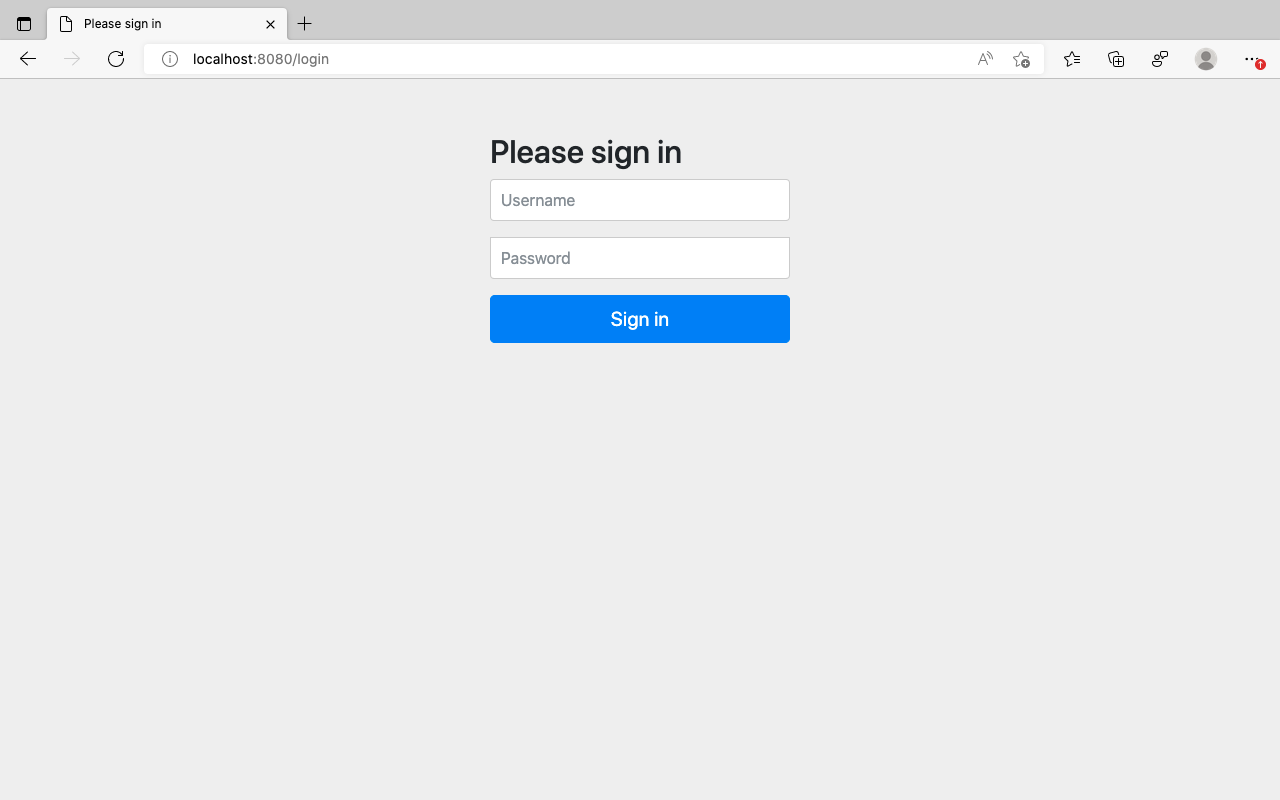
How do we access the APIs?
Spring Security creates an in-memory user with username user and a randomly generated password which can be found in the console:
Using generated security password: fdea9fea-3b53-43f8-b78b-046ca27b03af
This generated password is for development use only. Your security configuration must be updated before running your application in production.
This password changes each time we re-run the application. If we enter the credentials in the login form, we will be redirected to the requested API URL where we can see the list of courses.
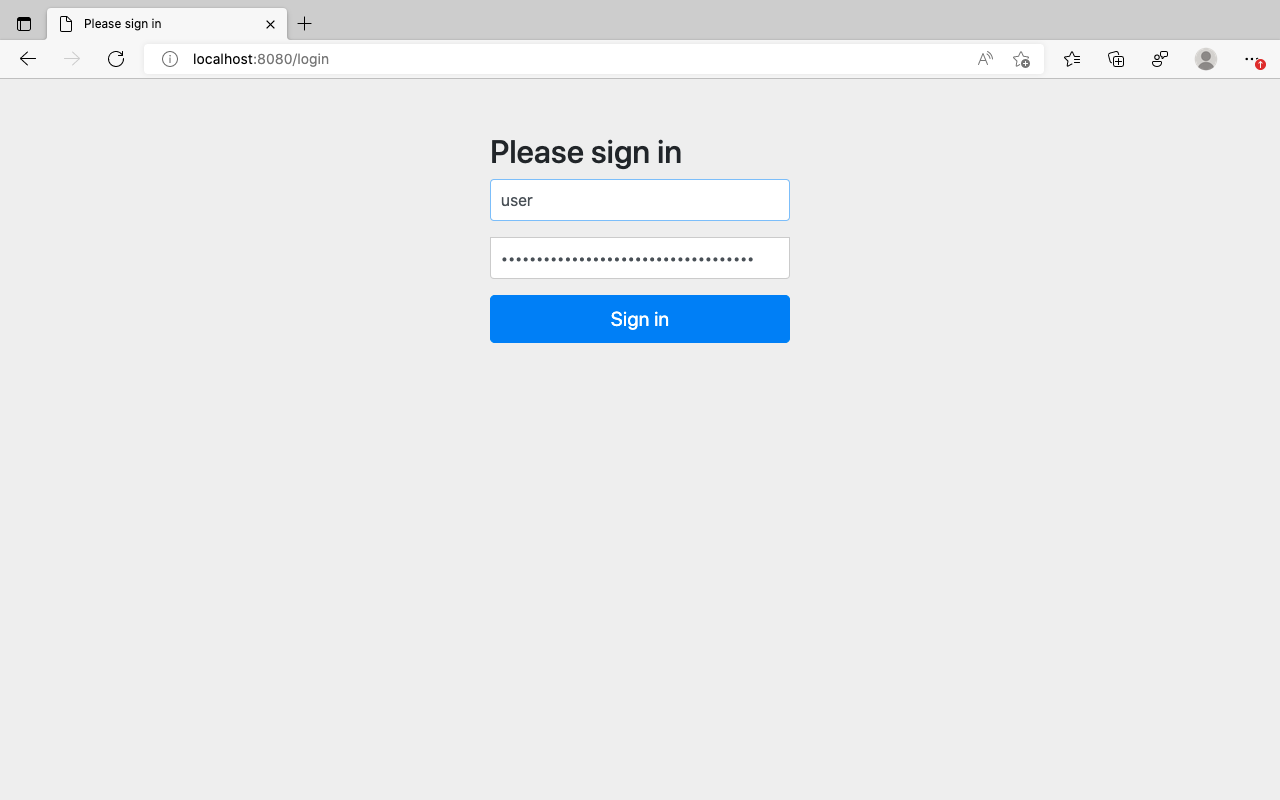
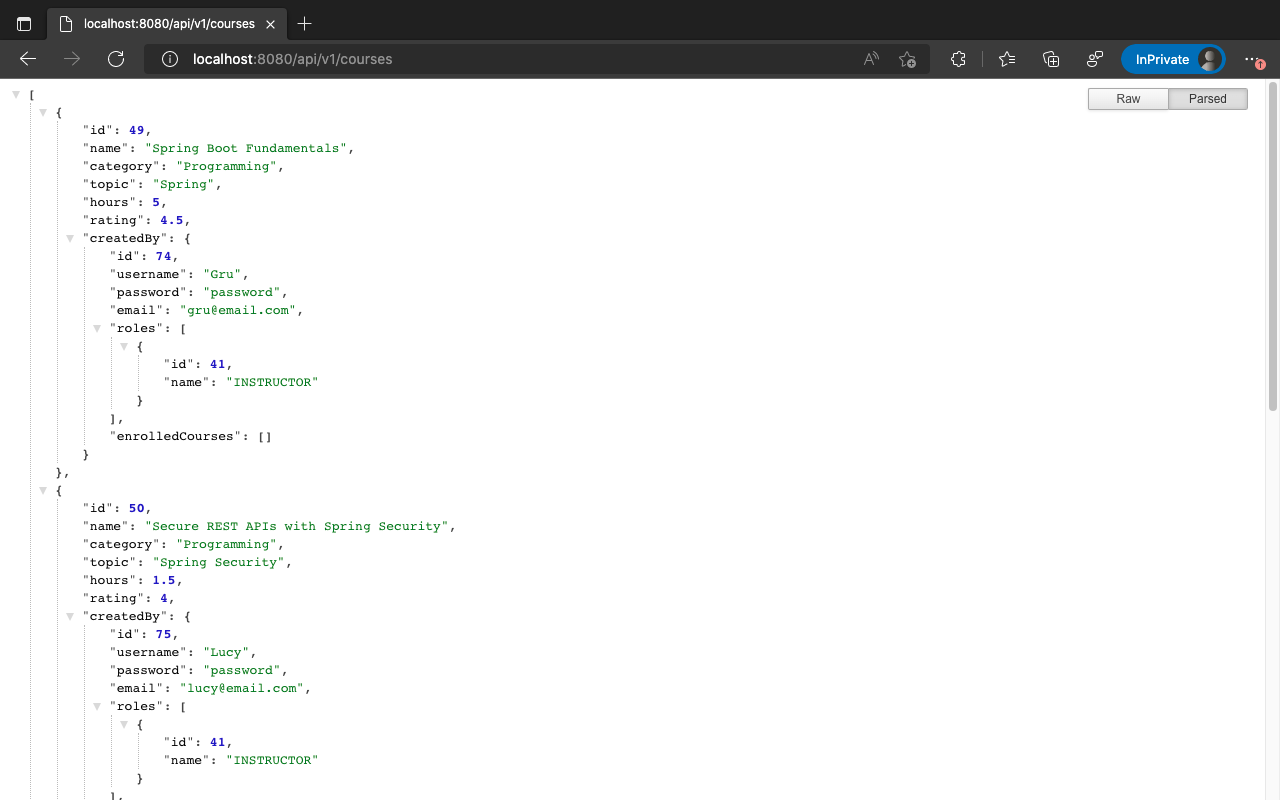
Similarly let's go back to Postman, choose Basic Auth in Authorization tab, and enter the same credentials, we will receive the list of courses as API response.
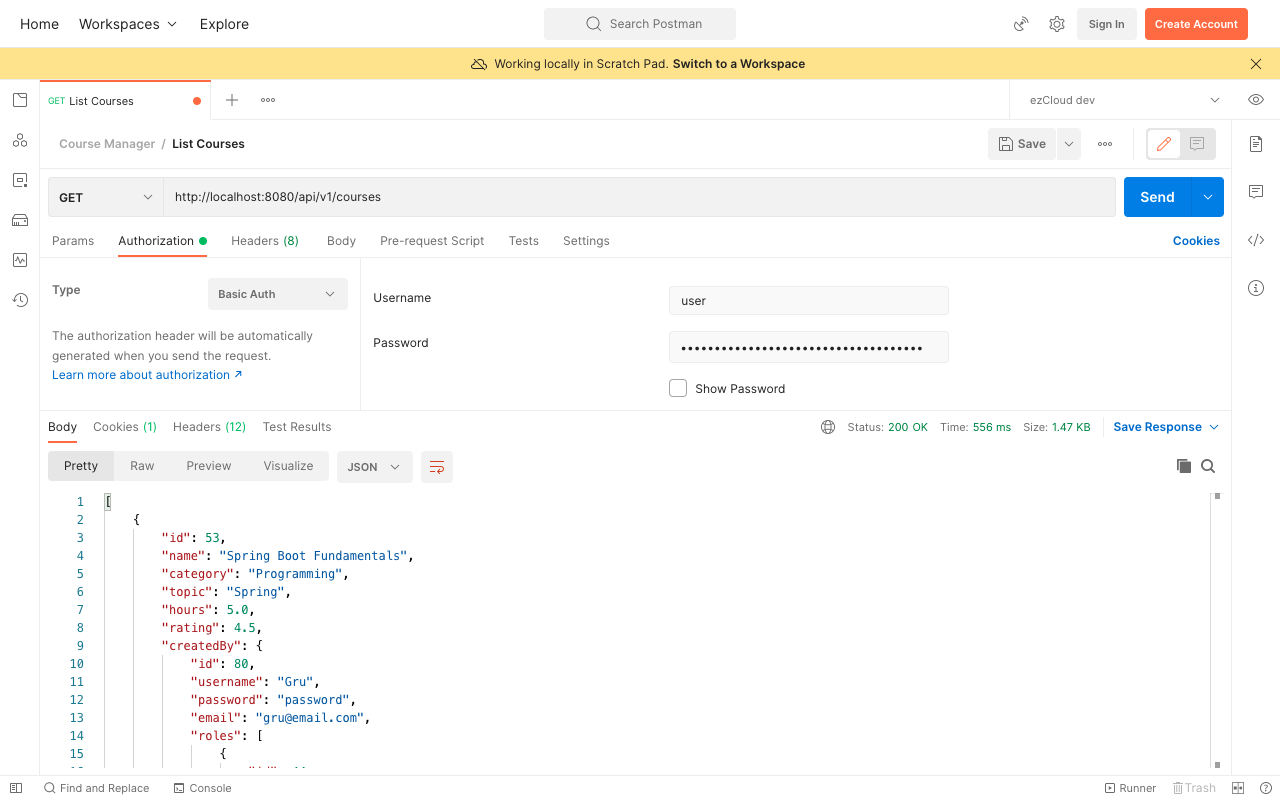
We can notice Spring Security behaves differently while accessing the API from Postman and Browser. This is because of different AuthenticationEntryPoint implementations used to start the authentication process.
Basic Auth is the out-of-the-box authentication mechanism enabled by Spring Security. And therefore BasicAuthenticationEntryPoint is the default AuthenticationEntryPoint. It responds with 401 Unauthorized error with WWW-Authenticate header value Basic realm="Realm" for any unauthenticated requests. This header value enabled us to know the authentication method used to access the resource while using Postman.
Whereas, if the unauthenticated request has any of the following Accept header values: text/html, application/xml, application/xhtml+xml, Spring Security will then replace the default AuthenticationEntryPoint with LoginUrlAuthenticationEntryPoint. It responds with 302 redirect error and redirects the browser to the default login page provided by Spring Security. Thus Basic Auth is replaced with form based login authentication mechanism while using browser.