Learn Spring Security
Contents
Permit Public APIs
We are now aware that the default behaviour of Spring Security secures all the resources. In addition we have configured HttpSecurity to authenticate any HTTP requests using Basic Auth. This enforced us to provide username and password everytime we access an API in order to authenticate ourselves, failing to provide them resulted in 401 Unauthorized error.
However, ListCourses and GetCourse APIs are open for anyone to access without authentication. In this chapter we will relax the access restriction for these APIs using antMatchers().
First let's add the Public API urls in a constant class like below:
public class SecurityConstants {
public static final String API_LIST_COURSES = "/api/v1/courses";
public static final String API_GET_COURSE = "/api/v1/courses/*";
public static final String[] PUBLIC_API_LIST = new String[] {
API_LIST_COURSES,
API_GET_COURSE
};
};
AntMatchers
antMatchers() are overloaded methods to configure restrictions for specific urls matching the provided antPattern urls. We can either chain them together with authorizeRequests() or pass it as an argument to the authorizeRequests() in the form of lambda expression.
Here we are going to use one of the overloaded methods which accepts both HttpMethod and antPattern urls: antMatchers(HttpMethod, String...)
@Configuration
public class ApiSecurityConfig {
@Bean
public SecurityFilterChain apiFilterChain(HttpSecurity http) throws Exception {
http
.authorizeRequests(auth -> auth
.antMatchers(GET, PUBLIC_API_LIST).permitAll()
.anyRequest().authenticated()
)
.httpBasic();
return http.build();
}
}
As ListCourses and CreateCourse endpoints are the same but with different HttpMethod, we have to specify HttpMethod.GET in the antMatchers to permit only the ListCourses API.
The order in which the antMatchers are defined is important, with most restricted resources defined at the top followed by least restricted resources at the bottom.
Test Public APIs
Restart the application and access the two Public APIs from Postman by selecting No Auth option in the Authorization tab. With No Auth we are not required to provide username and password. And we can notice the APIs returning the expected response with no authentication required anymore.
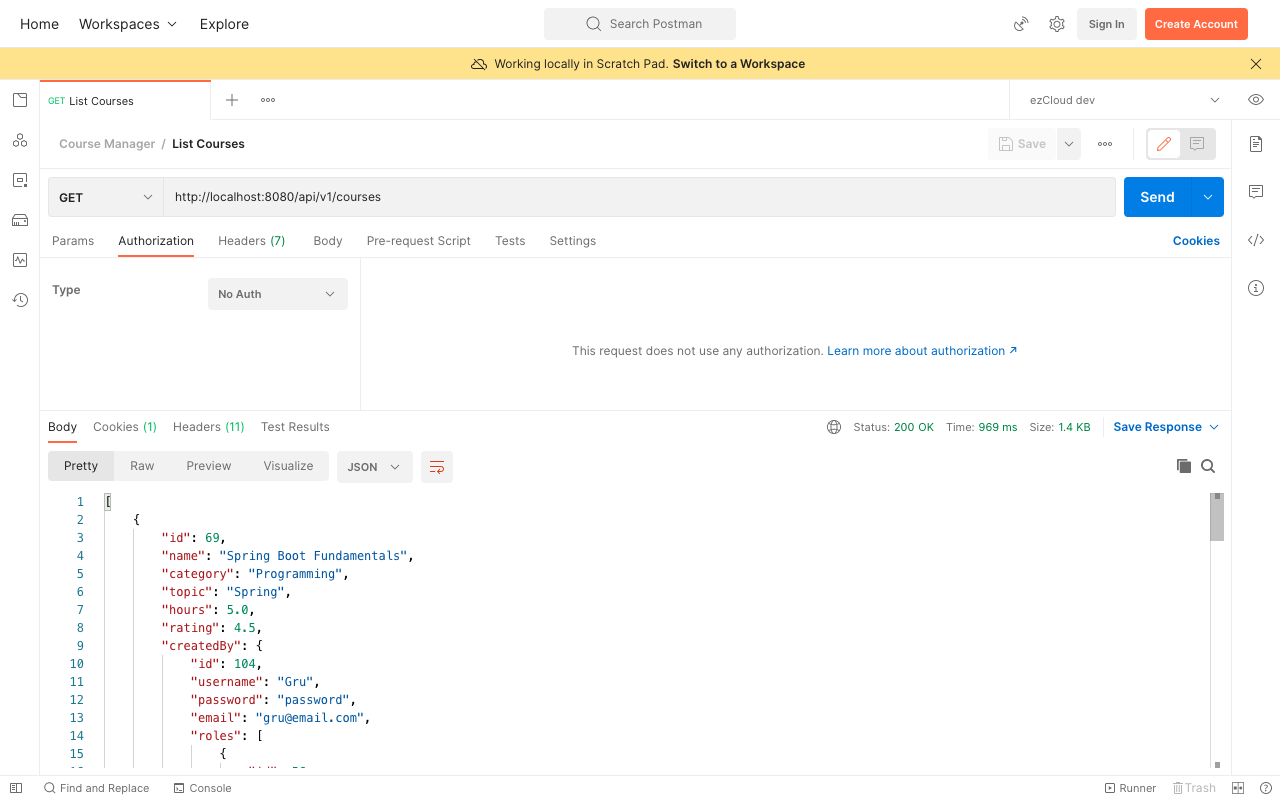